Vue-day06
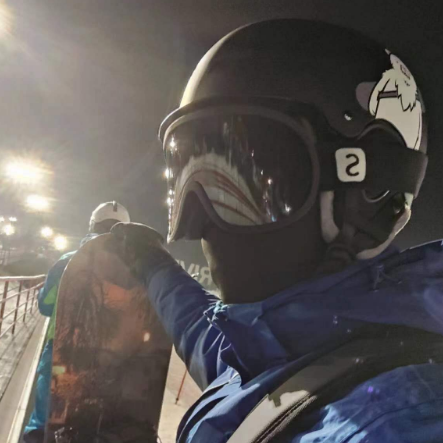
day06
VueRouter 的介绍
目标:认识插件 VueRouter ,掌握 VueRouter 的基本使用步骤
作用:修改地址栏路径时,切换显示匹配的组件
说明:Vue 官方的一个路由插件,是一个第三方包
VueRouter 的 使用(5+2)
5 个基础步骤(固定)
下载: 下载 VueRouter 模块到当前工程 v3.6.5
1
yarn add vue-router@3.6.5
引入
1
import VueRouter from 'vue-router'
安装注册 Vue.use(Vue 插件)
1
Vue.use(VueRouter) // VueRouter插件初始化
创建路由对象
1
2
3
4
5
6
7
8
9const router = new VueRouter({
// routes 路由规则们
// route 一条路由规则 { path: 路径, component: 组件 }
routes: [
{ path: '/find', component: Find },
{ path: '/my', component: My },
{ path: '/friend', component: Friend }
]
})注入到 new Vue 中,建立关联
1
2
3
4
5new Vue({
render: h => h(App),
router //简写!
}).$mount('#app')
2 个核心步骤:
创建需要的组件(views 目录),配置路由规则
1
2
3
4
5routes: [
{ path: '/find', component: Find },
{ path: '/my', component: My },
{ path: '/friend', component: Friend }
]配置导航,配置路由出口(路径匹配的组件显示的位置)
1
2
3
4
5
6
7
8
9<div id="app">
<div class="link">
/*对应匹配的跳转*/
<router-link to="/home">首页</router-link>
<router-link to="/search">搜索页</router-link>
</div>
/*出口位置*/
<router-view></router-view>
</div>
声明式导航 - 导航连接 (就是 router-link)
需求:实现导航高亮效果
vue-router 提供了一个全局组件 router-link(取代 a 标签)
- 能跳转,配置 to 属性指定路径(必须)。本质还是 a 标签,to 无需 #
- 能高亮,默认就会提供高亮类名,可以之间设置高亮样式
声明式导航 - 两个类名
说明:我们发现 router-link 自动给当前导航加了 两个高亮类名
router-link-active 模糊匹配(用的多)
to =”/my” 可以匹配 /my /my/a /my/b …
router-link-exact-active 精确匹配
to =”/my” 仅可以匹配 /my
这个两个高亮类名可以 自定义
1 | const router = new VueRouter({ routes: [...], linkActiveClass:'类名1', |
声明式导航 - 跳转传参
目标:在跳转路由时,进行传值
查询参数传参(比较适合传多个参数)
语法格式如下
to="/path?参数名=值&参数名2=值2"
对应页面组件接收传递过来的值
$router.query.参数名"
动态路由传参(优雅简洁,传单个参数比较方便)
配置动态路由
1
2
3
4
5
6const router = new VueRouter({
routes: [
{ path: '/home', component: Home },
{ path: '/search/:words', component: Search }
]
})配置导航连接
to="/path/参数值"
对应页面组件接收传递过来的值
$route.params.参数名"
动态路由参数可选符
/search/:words 表示,必须要传参数。如果不传参数,也希望匹配,可以加个可选符”?“
Vue 路由 - 重定向
说明:重定向 → 匹配 path 后,强制跳转 path 路径
语法:{path:匹配路径,redirect:重定向到的路径},
1 | const router = new VueRouter({ routes: [ { path: '/', redirect: '/home' }, { |
Vue 路由 - 404
作用:当路径找不到匹配时,给个提示页面
位置:配在路由最后
语法:path:”*“(任意路径) - 前面不匹配就命中最后这个
Vue 路由 - 模式设置
hash 路由(默认) 例如:http://loachost:8080/#/home
history 路由(常用) 例如:http://loachost:8080/home (以后上线需要服务器端支持)
1 | const router = new VueRouter({ |
编程式导航 - 基本跳转
编程式导航用 js 代码来进行跳转
两种语法:
path 路径跳转
1
2
3
4goSearch() { // 1. 通过路径的方式跳转 // (1) this.$router.push("路由路径")
【简写】 // this.$router.push("/search"); // (2) this.$router.push({
【完整写法】 // path:'路由路径' // }) this.$router.push({ path: "/search",
});name 命名路由跳转(适合 path 路径长的场景)
1
2
3this.$router.push({
name: "search",
});1
2// router.js 里面的代码
{ name: 'search', path: '/search/:words?', component: Search },
编程式导航 - 路由传参
两种跳转方式,对于两种传参方式都支持:
path 路径跳转传参
① query 传参
1 | this.$router.push("/路径?参数名1=参数值1&参数名2=参数值2"); |
② 动态路由传参(需要配动态路由)
1 | this.$router.push(/路径/参数值) |
name 命名路由跳转
① query 传参
1
2
3
4
5
6this.$router.push({
name: "路由名字",
query: {
参数名1: '参数值1',
参数名2: '参数值2',
},② 动态路由传参(需要配动态路由)
1
2
3
4
5this.$router.push({
name: "路由名字",
params: {
参数名1: '参数值1',
},选择原则:
第一步:先看路径,路径简单用 path,路径长就用 name,
第二步:然后参数多就选 query,单个参数用 params 。
- 标题: Vue-day06
- 作者: Hong Bing
- 创建于 : 2024-03-04 09:46:46
- 更新于 : 2024-03-04 09:49:14
- 链接: https://iyfhongbing.gitee.io/2024/03/04/Vue-day06/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。