Vue-day05
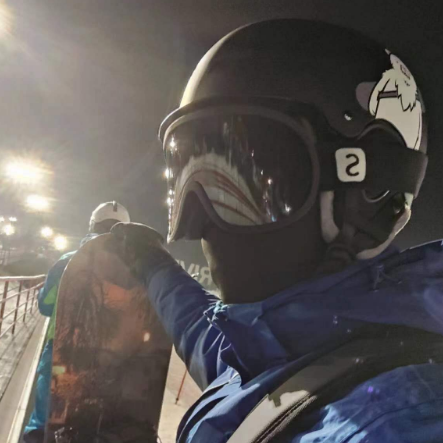
day 05
自定义指令:自己定义的指令,可以封装一些 dom 操作,扩展额外功能
需求:当页面加载时,让元素将获得焦点 (autofocus 在 safari 浏览器有兼容性)
操作 dom:dom 元素.focus()
1 | // 👇这是原来的方法 |
全局注册-语法
1 | // 全局注册指令 |
局部注册-语法
1 | directives: { |
使用:<input **v-指令名** type=”text”>
1 | <input v-focus type="text" /> |
自定义指令 - 指令的值
需求:实现一个 color 指令 - 传入不同的颜色,给标签设置文字颜色
语法:在绑定指令时,可以通过 ‘等号’ 的形式为指令 绑定 具体的参数值
1 | <h1 v-color="color1">指令赋值A</h1> |
通过 binding.value 可以拿到指令值,指令值修改会 触发 update 函数。
1 | directives: { |
插槽 - 默认插槽
插槽基本语法:
组件内需要定制的结构部分,改用
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17<template>
<div class="dialog">
<div class="dialog-header">
<h3>友情提示</h3>
<span class="close">✖️</span>
</div>
<div class="dialog-content">
<!-- 1. 在需要定制的地方使用slot占位 🍳 -->
<slot></slot>
</div>
<div class="dialog-footer">
<button>取消</button>
<button>确认</button>
</div>
</div>
</template>使用组件时,
标签内部,传入结构替换 slot 1
2<MyDialog>你确认吗?</MyDialog>
<MyDialog><div>你确认要退出本系统么?</div></MyDialog>
插槽 - 后备内容(默认值)
插槽后备内容:封装组件时,可以为预留的 <slot>
插槽提供后备内容(默认内容)。
语法:在
效果:
外部使用组件时,不传东西,则 slot 会显示后备内容
1 | <MyDialog></MyDialog> |
外部使用组件时,传东西了,则 slot 整体会被换掉
1 | <MyDialog><div>你确认要退出本系统么?</div></MyDialog> |
组件代码这样写:
1 | <template> |
插槽 - 具名插槽
具名插槽简化语法:
多个 slot 使用 name 属性区分名字
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16<template>
<div class="dialog">
<div class="dialog-header">
<slot name="head"></slot>
<span class="close">✖️</span>
</div>
<div class="dialog-content">
<!-- 在slot内写的东西可以做为默认内容显示 -->
<slot name="content">我就是默认文本</slot>
</div>
<div class="dialog-footer">
<slot name="footer"></slot>
</div>
</div>
</template>template 配合 v-slot: 名字来分发对应标签
1
2
3
4
5
6
7
8
9
10
11
12<MyDialog>
<template v-slot:head>
<div>我是标题</div>
</template>
<template #content>
<div>我是内容</div>
</template>
<template #footer>
<button>确认</button>
<button>取消</button>
</template>
</MyDialog>v-slot:插槽名
可以简化成#插槽名
插槽 - 作用域插槽
基本使用步骤:
给 slot 标签,以 添加属性的方式传值
1
2
3// 这里不叫row 叫别的也行
<slot :row="item" msg="非变量测试文本"></slot>所有添加的属性,都会被收集到一个对象中
1
2
3
4
5{
row:{id:1,name:'smm',age:19},
msg:'非变量测试文本'
}在 template 中,通过
#插槽名=“obj”
接收, 默认插槽名为 default1
2
3
4
5
6<MyTable :data="list">
<!-- 3. 通过template #插槽名=“变量名” 接收 -->
<template #default="obj">
<button @click="delFn(obj.row.id)">删除</button>
</template>
</MyTable>
- 标题: Vue-day05
- 作者: Hong Bing
- 创建于 : 2024-03-04 09:44:36
- 更新于 : 2024-03-04 09:45:20
- 链接: https://iyfhongbing.gitee.io/2024/03/04/Vue-day05/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。
评论